Pure C++ 20 - Raw & Unfiltered
Pure C++ 20 - Raw & Unfiltered
![Pure C++ 20 - Raw & Unfiltered]()
What you'll learn
Learn about the new C++20 language standard features
Migrate existing C++ codebase to C++20
Use coroutines to write concurrent code
Use modules instead of headers to modularize software
Use ranges library to simplify working with containers & algorithms
Use concepts to apply constraints on algorithms & classes
Requirements
Basic understanding of C++11
Description
This course only focuses on the new C++20 standard features without going into the basics or details of previous standards of C++ or basics of object-oriented programming. You must've some basic understanding of C++11 in order to follow through in this course. In case, you're not familiar with modern C++ features, check out my other course called Complete Modern C++.This course will aim to explain the new core language & the library features. You'll learn to use designated initializers, consteval, consinit, unevaluated constext usage of lambda expressions, enum usage, etc. You'll also get to know about the new way through which C++ code is reused without using header files. This is possible through modules, which is a faster and easier way to reuse existing functions or classes.If you implement classes for use with containers, then you'll have to provide implementation of many comparison operators which becomes tedious and error-prone. C++20 provides a new operator called the three-way comparison operator (space-ship operator) that simplifies implementation of comparison operators. Additionally, it now becomes easier to use algorithms with containers through ranges. No need to use begin() & end() functions to operate on the container.Concepts is a new way of applying constraints on template arguments. It is much simpler to use as compared to SFINAE (enable_if). Learn & implement concepts to ensure your functions accept the right kind of arguments and avoid runtime errors.Finally, you'll learn about resumable functions, called coroutines. Using coroutines, you can write concurrent code without the need for synchronization or multiple threads. This enables you to write high performance code without the overhead of creating & destroying threads.If you're ready, then let's get started right away.
Overview
Section 1: Introduction to C++20
Lecture 1 Introduction
Lecture 2 Compiler support
Lecture 3 Source Code
Section 2: Core Language Changes
Lecture 4 Source Code
Lecture 5 Designated Initializers - I
Lecture 6 Designated Initializers - II
Lecture 7 Designated Initializers - III
Lecture 8 Range-based For Loop - I
Lecture 9 Range-based For Loop - II
Lecture 10 UTF-8 - I
Lecture 11 UTF-8 - II
Lecture 12 Structured Bindings
Lecture 13 Constant Expressions - constexpr
Lecture 14 Immediate Functions - consteval
Lecture 15 Compiled-time Initialization - constinit
Lecture 16 explicit bool - I
Lecture 17 explicit bool - II
Lecture 18 explicit bool - III
Lecture 19 Non-Type Template Parameters
Section 3: Lambda Expressions
Lecture 20 Source Code
Lecture 21 implicit this
Lecture 22 Template Parameters - I
Lecture 23 Template Parameters - II
Lecture 24 Lambda In Unevaluated Context
Section 4: More Core Language Changes
Lecture 25 Attributes - I
Lecture 26 Attributes - II
Lecture 27 enums
Section 5: Concepts
Lecture 28 Source Code
Lecture 29 Constraining Template Arguments
Lecture 30 Concepts Introduction
Lecture 31 Concepts Implementation
Lecture 32 Concepts Usage Types
Lecture 33 Abbreviated Templates
Lecture 34 Requirement Types - I
Lecture 35 Requirement Types - II
Lecture 36 Combining Requirements
Lecture 37 More Examples
Section 6: Modules
Lecture 38 Source Code
Lecture 39 C++ Build Process Overview
Lecture 40 Introduction to Modules
Lecture 41 Modules Example
Lecture 42 Module Naming
Lecture 43 Modules Build Process
Lecture 44 Declaration & Definition Separation
Lecture 45 Submodules
Lecture 46 Partition Modules
Lecture 47 Header Units
Lecture 48 Module Private Marker
Section 7: Three-way Comparison
Lecture 49 Source Code
Lecture 50 Comparison Operators - I
Lecture 51 Comparison Operators - II
Lecture 52 Three-way Comparison Operator - I
Lecture 53 Three-way Comparison Operator - II
Lecture 54 Three-way Comparison Operator - III
Lecture 55 Three-way Comparison Operator - IV
Lecture 56 Synthesized Expressions - I
Lecture 57 Synthesized Expressions - II
Lecture 58 Comparisons
Lecture 59 Equality Operator - I
Lecture 60 Equality Operator - II
Lecture 61 Equality Operator - III
Lecture 62 Ordering Categories
Lecture 63 Strong Ordering
Lecture 64 Weak Ordering - I
Lecture 65 Weak Ordering - II
Lecture 66 Partial Ordering - I
Lecture 67 Partial Ordering - II
Lecture 68 Ordering Summary
Lecture 69 Backward Compatibility
Lecture 70 Utilities - I
Lecture 71 Utilities - II
Lecture 72 Utilities - III
Lecture 73 Utilities - IV
Lecture 74 Summary
Section 8: Ranges
Lecture 75 Source Code
Lecture 76 Algorithms & Iterators
Lecture 77 Ranges Introduction
Lecture 78 Ranges Example
Lecture 79 Range Concepts - I
Lecture 80 Range Concepts - II
Lecture 81 Range Concepts - III
Lecture 82 Projections - I
Lecture 83 Projections - II
Lecture 84 Projections - III
Lecture 85 Algorithm Return Types - I
Lecture 86 Algorithm Return Types - II
Lecture 87 Algorithm Return Types - III
Lecture 88 Views - I
Lecture 89 Views - II
Lecture 90 Views - III
Section 9: Coroutines
Lecture 91 Source code
Lecture 92 Subroutines
Lecture 93 Coroutines Introduction
Lecture 94 Coroutines Example
Lecture 95 Coroutine Anatomy
Lecture 96 Coroutine Implementation
Lecture 97 Coroutine Workflow - I
Lecture 98 Coroutine Workflow - II
Lecture 99 Coroutine Workflow - III
Lecture 100 Returning Values (co_return)
Lecture 101 Generator (co_yield)
Lecture 102 Awaitable
Lecture 103 Awaitable implementation
Lecture 104 Awaiter
Lecture 105 Awaiter Implementation - I
Lecture 106 Awaiter Implementation - II
Lecture 107 Awaiter Methods
Lecture 108 Coroutine Concurrency - I
Lecture 109 Coroutine Concurrency - II
Lecture 110 Coroutine Concurrency - III
Lecture 111 Exceptions in Coroutines
Lecture 112 Initialization Stage Exceptions
Lecture 113 Execution Stage Exceptions - I
Lecture 114 Execution Stage Exceptions - II
Lecture 115 Conclusion
C++ developers who want to learn C++20,C++ developers who want to migrate their code to C++20
![Pure C++ 20 - Raw & Unfiltered]()
Say "Thank You"
rapidgator.net:
https://rapidgator.net/file/09af7be2a95928f01c362e24d6ae23c9/jrcll.Pure.C.20..Raw..Unfiltered.part1.rar.html
https://rapidgator.net/file/1937342485d897932cff1d3f769f5f49/jrcll.Pure.C.20..Raw..Unfiltered.part2.rar.html
https://rapidgator.net/file/e8465dda385b2a4ad0c470769e8e7662/jrcll.Pure.C.20..Raw..Unfiltered.part3.rar.html
https://rapidgator.net/file/c9138ae64230d35f0ac0be747ca4a38a/jrcll.Pure.C.20..Raw..Unfiltered.part4.rar.html
ddownload.com:
https://ddownload.com/qsa9he4ss5h6/jrcll.Pure.C.20..Raw..Unfiltered.part1.rar
https://ddownload.com/1yr9b5e9sar6/jrcll.Pure.C.20..Raw..Unfiltered.part2.rar
https://ddownload.com/17h5nr6uyesl/jrcll.Pure.C.20..Raw..Unfiltered.part3.rar
https://ddownload.com/yvxtlmwfltsh/jrcll.Pure.C.20..Raw..Unfiltered.part4.rar
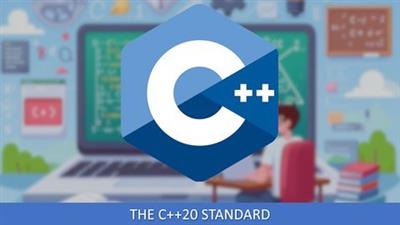
Published 11/2024
MP4 | Video: h264, 1920x1080 | Audio: AAC, 44.1 KHz
Language: English | Size: 3.77 GB | Duration: 9h 42m
C++20 without the fluff and baggage of old C++
MP4 | Video: h264, 1920x1080 | Audio: AAC, 44.1 KHz
Language: English | Size: 3.77 GB | Duration: 9h 42m
C++20 without the fluff and baggage of old C++
What you'll learn
Learn about the new C++20 language standard features
Migrate existing C++ codebase to C++20
Use coroutines to write concurrent code
Use modules instead of headers to modularize software
Use ranges library to simplify working with containers & algorithms
Use concepts to apply constraints on algorithms & classes
Requirements
Basic understanding of C++11
Description
This course only focuses on the new C++20 standard features without going into the basics or details of previous standards of C++ or basics of object-oriented programming. You must've some basic understanding of C++11 in order to follow through in this course. In case, you're not familiar with modern C++ features, check out my other course called Complete Modern C++.This course will aim to explain the new core language & the library features. You'll learn to use designated initializers, consteval, consinit, unevaluated constext usage of lambda expressions, enum usage, etc. You'll also get to know about the new way through which C++ code is reused without using header files. This is possible through modules, which is a faster and easier way to reuse existing functions or classes.If you implement classes for use with containers, then you'll have to provide implementation of many comparison operators which becomes tedious and error-prone. C++20 provides a new operator called the three-way comparison operator (space-ship operator) that simplifies implementation of comparison operators. Additionally, it now becomes easier to use algorithms with containers through ranges. No need to use begin() & end() functions to operate on the container.Concepts is a new way of applying constraints on template arguments. It is much simpler to use as compared to SFINAE (enable_if). Learn & implement concepts to ensure your functions accept the right kind of arguments and avoid runtime errors.Finally, you'll learn about resumable functions, called coroutines. Using coroutines, you can write concurrent code without the need for synchronization or multiple threads. This enables you to write high performance code without the overhead of creating & destroying threads.If you're ready, then let's get started right away.
Overview
Section 1: Introduction to C++20
Lecture 1 Introduction
Lecture 2 Compiler support
Lecture 3 Source Code
Section 2: Core Language Changes
Lecture 4 Source Code
Lecture 5 Designated Initializers - I
Lecture 6 Designated Initializers - II
Lecture 7 Designated Initializers - III
Lecture 8 Range-based For Loop - I
Lecture 9 Range-based For Loop - II
Lecture 10 UTF-8 - I
Lecture 11 UTF-8 - II
Lecture 12 Structured Bindings
Lecture 13 Constant Expressions - constexpr
Lecture 14 Immediate Functions - consteval
Lecture 15 Compiled-time Initialization - constinit
Lecture 16 explicit bool - I
Lecture 17 explicit bool - II
Lecture 18 explicit bool - III
Lecture 19 Non-Type Template Parameters
Section 3: Lambda Expressions
Lecture 20 Source Code
Lecture 21 implicit this
Lecture 22 Template Parameters - I
Lecture 23 Template Parameters - II
Lecture 24 Lambda In Unevaluated Context
Section 4: More Core Language Changes
Lecture 25 Attributes - I
Lecture 26 Attributes - II
Lecture 27 enums
Section 5: Concepts
Lecture 28 Source Code
Lecture 29 Constraining Template Arguments
Lecture 30 Concepts Introduction
Lecture 31 Concepts Implementation
Lecture 32 Concepts Usage Types
Lecture 33 Abbreviated Templates
Lecture 34 Requirement Types - I
Lecture 35 Requirement Types - II
Lecture 36 Combining Requirements
Lecture 37 More Examples
Section 6: Modules
Lecture 38 Source Code
Lecture 39 C++ Build Process Overview
Lecture 40 Introduction to Modules
Lecture 41 Modules Example
Lecture 42 Module Naming
Lecture 43 Modules Build Process
Lecture 44 Declaration & Definition Separation
Lecture 45 Submodules
Lecture 46 Partition Modules
Lecture 47 Header Units
Lecture 48 Module Private Marker
Section 7: Three-way Comparison
Lecture 49 Source Code
Lecture 50 Comparison Operators - I
Lecture 51 Comparison Operators - II
Lecture 52 Three-way Comparison Operator - I
Lecture 53 Three-way Comparison Operator - II
Lecture 54 Three-way Comparison Operator - III
Lecture 55 Three-way Comparison Operator - IV
Lecture 56 Synthesized Expressions - I
Lecture 57 Synthesized Expressions - II
Lecture 58 Comparisons
Lecture 59 Equality Operator - I
Lecture 60 Equality Operator - II
Lecture 61 Equality Operator - III
Lecture 62 Ordering Categories
Lecture 63 Strong Ordering
Lecture 64 Weak Ordering - I
Lecture 65 Weak Ordering - II
Lecture 66 Partial Ordering - I
Lecture 67 Partial Ordering - II
Lecture 68 Ordering Summary
Lecture 69 Backward Compatibility
Lecture 70 Utilities - I
Lecture 71 Utilities - II
Lecture 72 Utilities - III
Lecture 73 Utilities - IV
Lecture 74 Summary
Section 8: Ranges
Lecture 75 Source Code
Lecture 76 Algorithms & Iterators
Lecture 77 Ranges Introduction
Lecture 78 Ranges Example
Lecture 79 Range Concepts - I
Lecture 80 Range Concepts - II
Lecture 81 Range Concepts - III
Lecture 82 Projections - I
Lecture 83 Projections - II
Lecture 84 Projections - III
Lecture 85 Algorithm Return Types - I
Lecture 86 Algorithm Return Types - II
Lecture 87 Algorithm Return Types - III
Lecture 88 Views - I
Lecture 89 Views - II
Lecture 90 Views - III
Section 9: Coroutines
Lecture 91 Source code
Lecture 92 Subroutines
Lecture 93 Coroutines Introduction
Lecture 94 Coroutines Example
Lecture 95 Coroutine Anatomy
Lecture 96 Coroutine Implementation
Lecture 97 Coroutine Workflow - I
Lecture 98 Coroutine Workflow - II
Lecture 99 Coroutine Workflow - III
Lecture 100 Returning Values (co_return)
Lecture 101 Generator (co_yield)
Lecture 102 Awaitable
Lecture 103 Awaitable implementation
Lecture 104 Awaiter
Lecture 105 Awaiter Implementation - I
Lecture 106 Awaiter Implementation - II
Lecture 107 Awaiter Methods
Lecture 108 Coroutine Concurrency - I
Lecture 109 Coroutine Concurrency - II
Lecture 110 Coroutine Concurrency - III
Lecture 111 Exceptions in Coroutines
Lecture 112 Initialization Stage Exceptions
Lecture 113 Execution Stage Exceptions - I
Lecture 114 Execution Stage Exceptions - II
Lecture 115 Conclusion
C++ developers who want to learn C++20,C++ developers who want to migrate their code to C++20
Screenshots
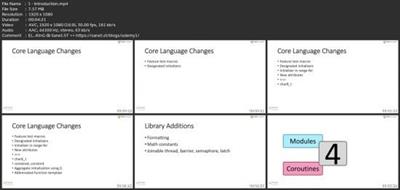
Say "Thank You"
rapidgator.net:
https://rapidgator.net/file/09af7be2a95928f01c362e24d6ae23c9/jrcll.Pure.C.20..Raw..Unfiltered.part1.rar.html
https://rapidgator.net/file/1937342485d897932cff1d3f769f5f49/jrcll.Pure.C.20..Raw..Unfiltered.part2.rar.html
https://rapidgator.net/file/e8465dda385b2a4ad0c470769e8e7662/jrcll.Pure.C.20..Raw..Unfiltered.part3.rar.html
https://rapidgator.net/file/c9138ae64230d35f0ac0be747ca4a38a/jrcll.Pure.C.20..Raw..Unfiltered.part4.rar.html
ddownload.com:
https://ddownload.com/qsa9he4ss5h6/jrcll.Pure.C.20..Raw..Unfiltered.part1.rar
https://ddownload.com/1yr9b5e9sar6/jrcll.Pure.C.20..Raw..Unfiltered.part2.rar
https://ddownload.com/17h5nr6uyesl/jrcll.Pure.C.20..Raw..Unfiltered.part3.rar
https://ddownload.com/yvxtlmwfltsh/jrcll.Pure.C.20..Raw..Unfiltered.part4.rar